OneSDK Implementation Guide
From document verification to fraud prevention, OneSDK handles the complexity of multiple vendor integrations while providing a unified API surface
This guide provides a conceptual overview of OneSDK integration with basic code examples. The code snippets shown are illustrative and need to be adapted into your specific framework and application architecture. For complete, working examples:
- See our Framework Integration Guides for Angular, React, Vue.js, Next.js and other frameworks
- Visit our GitHub repository for extensive sample code and reference implementations
- Check out our Hosted Implementation Examples for end-to-end solutions hosted by FrankieOne
Core Modules Overview
Complete KYC workflow for individual verification, including document handling, address verification, and profile management.
Advanced biometric verification capabilities including facial recognition, liveness detection, and matching.
Intelligent document scanning and data extraction for IDs, passports, and other verification documents.
Comprehensive identity document verification with support for multiple document types across jurisdictions.
Customizable electronic Know Your Customer forms with built-in validation and compliance checks.
Real-time fraud prevention and detection across multiple risk vectors.
OneSDK Modules Data Capture Flow
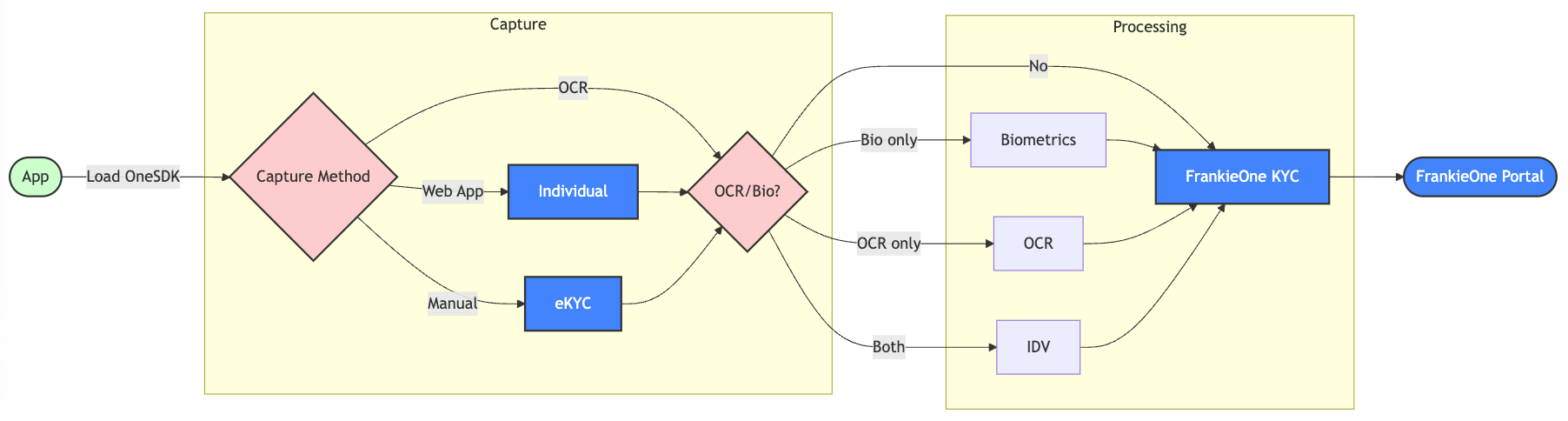
Quick Integration Guide
Implementation Best Practices
Error Handling
Module Coordination
Performance Optimization
Initialize modules only when needed and release resources after use. This is especially important for camera-based operations in the Biometrics and OCR modules.
Common Integration Scenarios
Basic KYC
Enhanced Verification
Each module can be used independently or as part of a comprehensive verification flow. Check individual module documentation for detailed implementation guidelines.
Content Security Policy (CSP) Settings
You may need to adjust your Content Security Policy (CSP) settings to allow vendors’ scripts and resources to load correctly. Below are the additional CSP rules required based on the vendor you are integrating with: