Retry
The Retry flow enables users to review and correct their submitted information when verification is partially successful. This feature improves verification success rates by allowing users to fix errors or provide additional information.
Flow Overview
Implementation
Smart Retry Behavior
Previous Address Request
Triggered when KYC check results in a partial match and the user hasn’t modified their personal details.
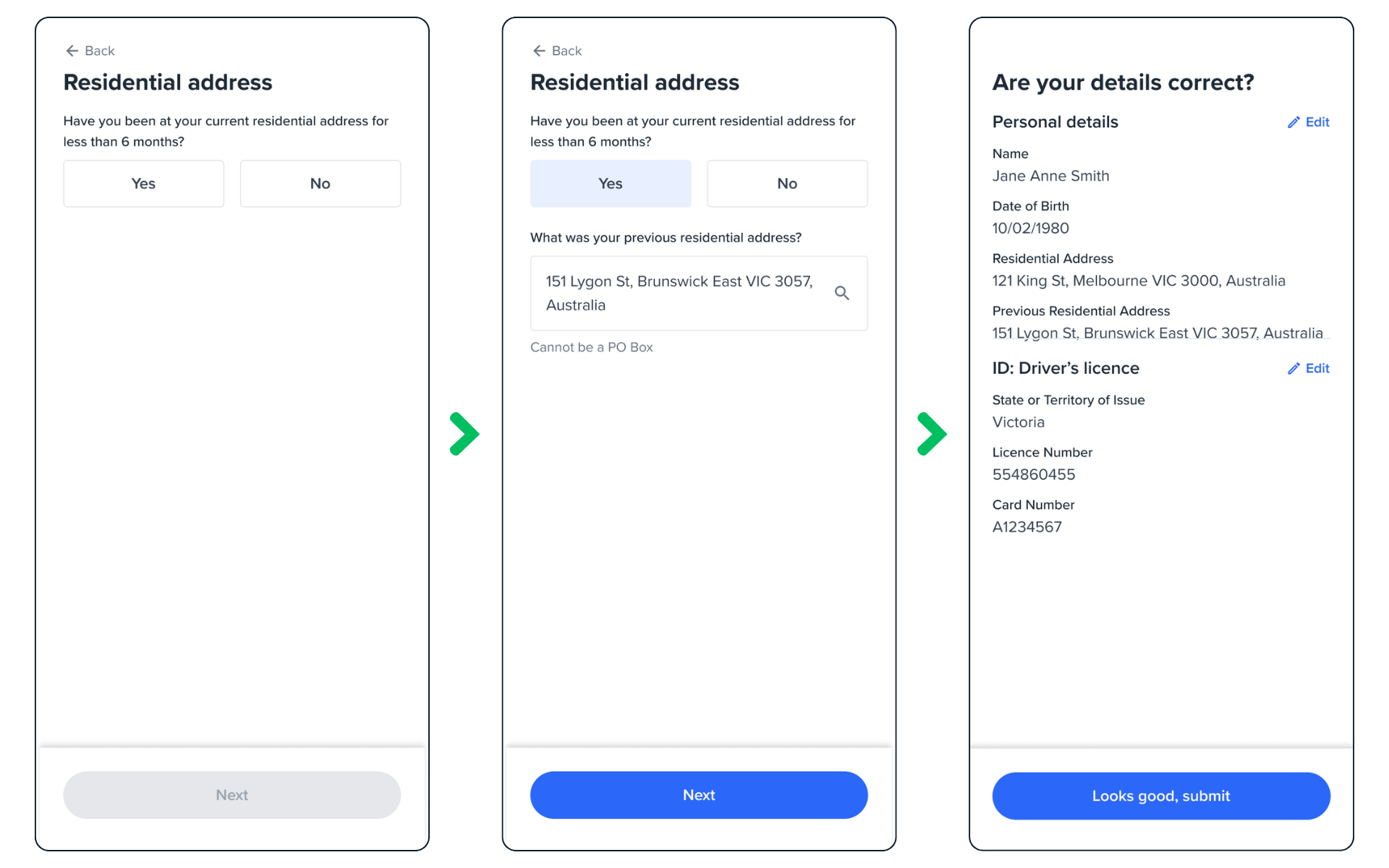
This flow:
- Detects partial KYC match
- Checks if personal details remained unchanged
- Prompts for previous address
- Uses additional data to improve verification chances
To customize the text for previous address request, override addresssI.fullAddress
field
Additional ID Request
Triggered when KYC passes but ID verification fails, and the user hasn’t modified their ID details.
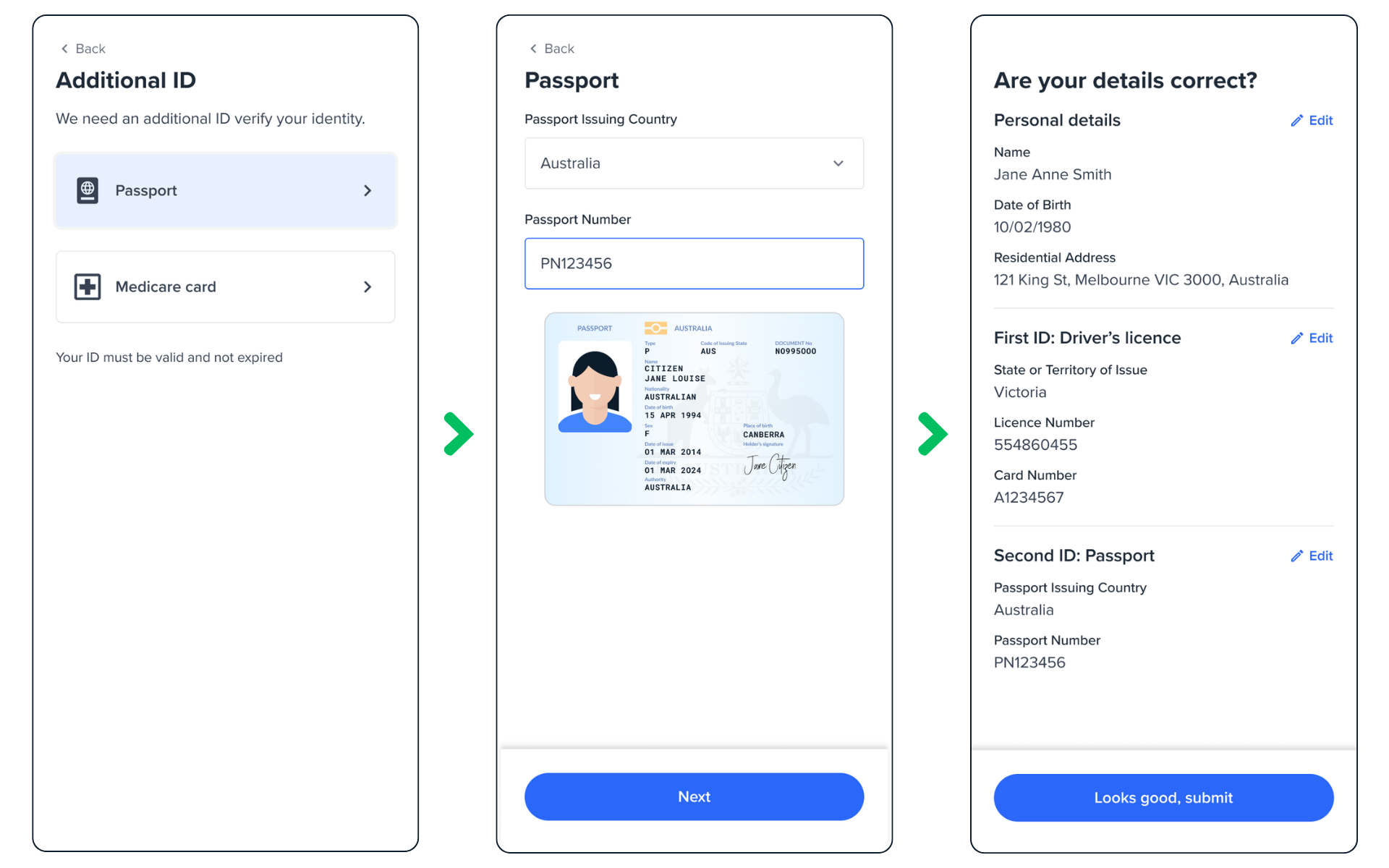
This flow:
- Detects successful KYC but failed ID verification
- Checks if ID details remained unchanged
- Prompts for alternative ID document
- Resubmits for verification
To customize the text for additional ID request, use additionInfo
property
Best Practices
Retry Limits
Implement a maximum retry count to prevent endless attempts:
User Experience
- Clear error messaging
- Intuitive navigation
- Progress indication
- Helpful prompts for additional information
Remember to handle edge cases such as: - Network failures during verification
- Timeout scenarios - Maximum retry limit reached - Invalid or corrupt document uploads